How to Learn Programming
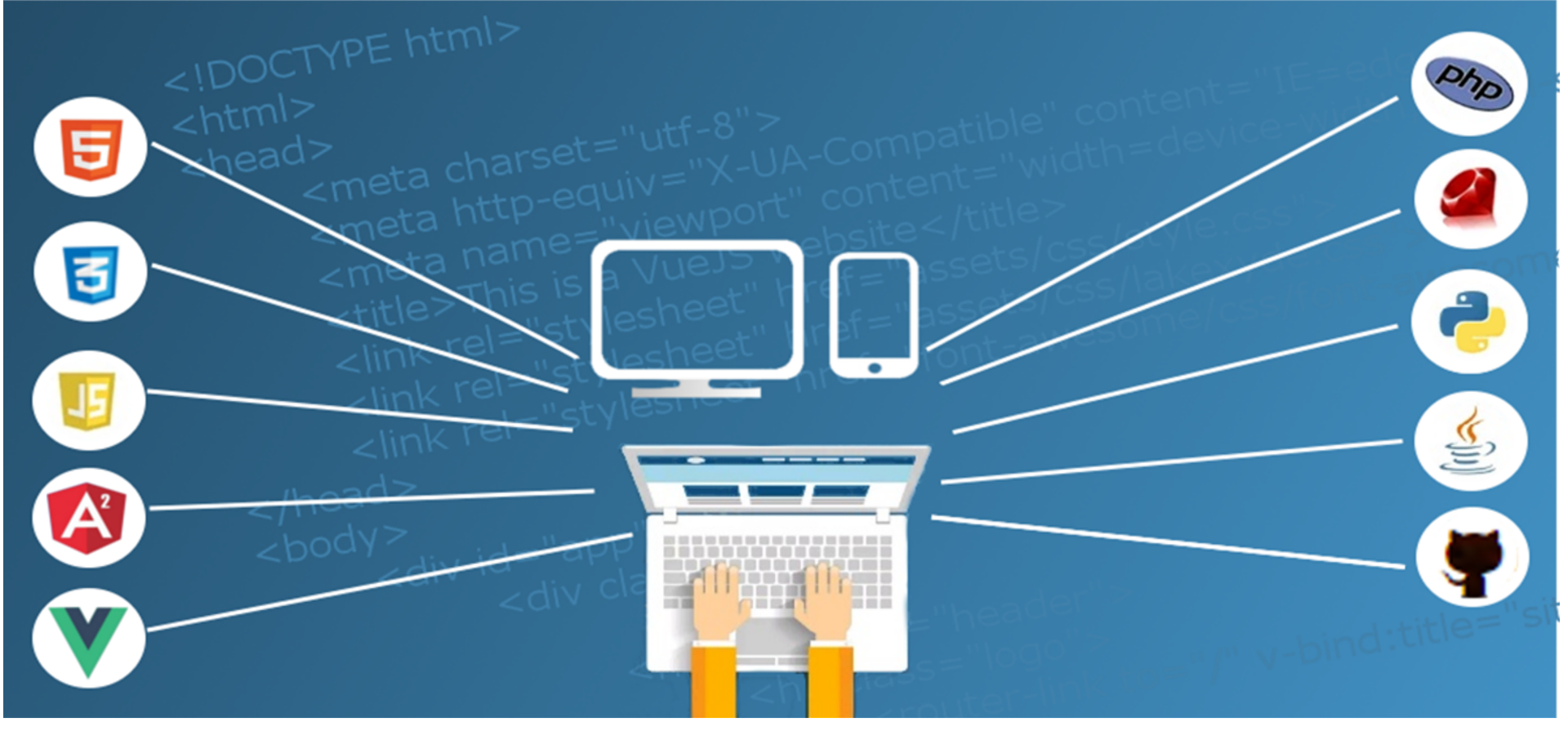
In the recent years, demand for software developers has exploded. The shortage of good developers has driven salaries and compensation to unprecedented levels.
This situation prompted many people to rethink their career choices and start learning programming. If you are among them, and wondering how to learn programming, you are in the right place.
In this post, I will give you a complete step-by-step guide on what you should learn and in what order to get your software development career of the ground.
How to learn programming (Complete step-by-step guide)
1. Learn theoretical fundamentals
2. Learn basic Linux usage
3. C as your first programming language
4. Basic data structures and algorithms
5. Object oriented programming with Java
6. Networking fundamentals
7. Database basics
8. Basic CI/CD concepts
9. Security best practices
10. Virtualization and cloud
1. Learn theoretical fundamentals
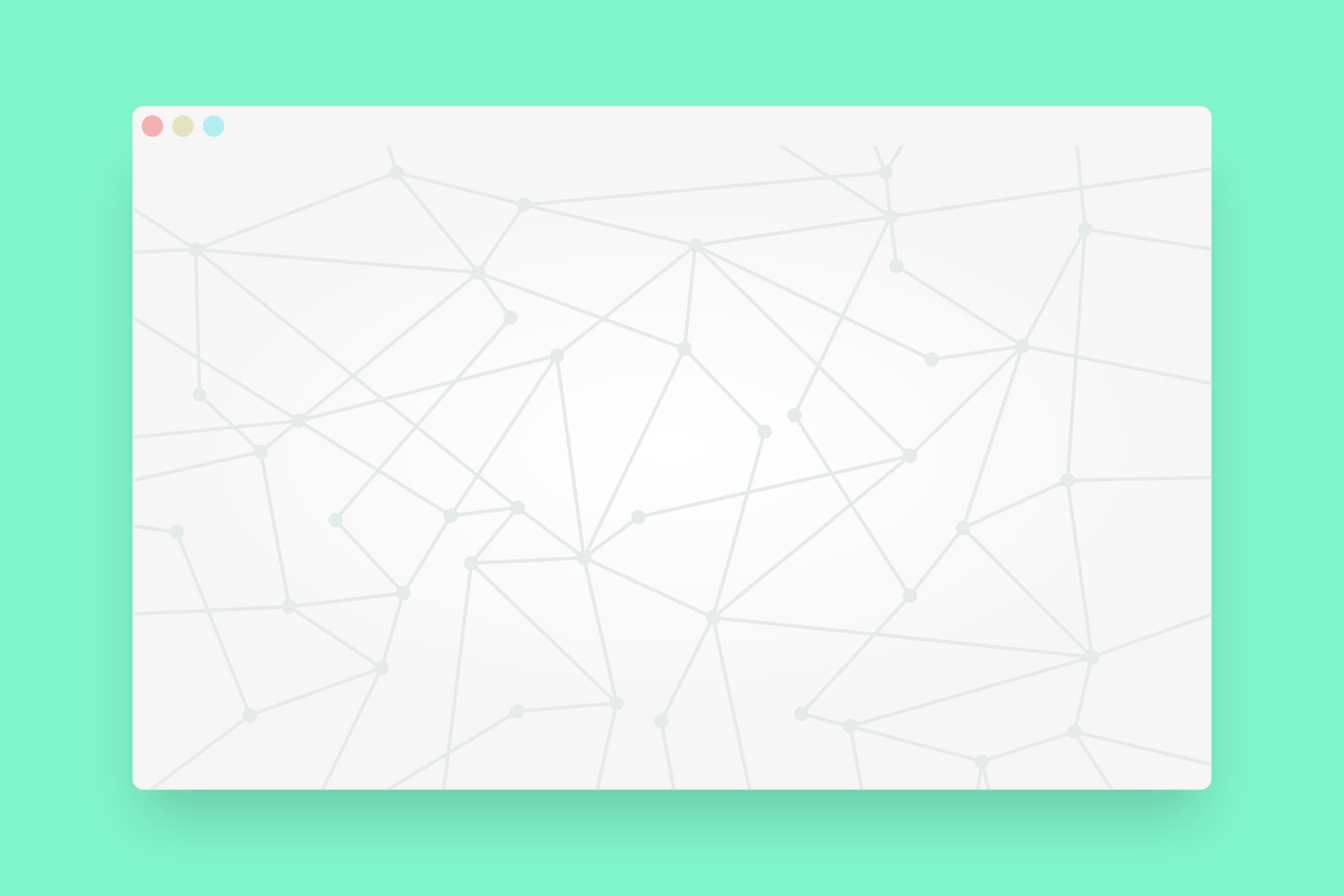
Learn programming fundamentals as the first step towards learning programming
When people who want to learn programming ask how they should start, the most common answer online is “just pick a language and start”. While this does not sound like a bad advice on the face of it, my opinion is that is only good for people who have had at least some exposure to programming and inner workings of the computer. For compete beginners, it’s a double edged sword.
The good side here is that it provides instant gratification. Anybody can whip up a “Hello, world” style program in a few minutes, and then they can say “Look, ma. I’m a programmer”.
On the flip side, this approach can frequently cause beginners to struggle when they start learning more advance concepts. They lack theoretical foundation on which programming is based.
Learning these foundations is the first things you should do when starting your programming journey. These foundation should cover the following:
Number systems - binary, octal, hexadecimal. You should be able to convert number between these 3 and decimal numbers. Learn basic operations with binary numbers, like addition and subtraction
Boolean algebra - this is theoretical base of digital electronics, and by extension, computing. You don’t need to go into details, but should have the knowledge of basic operations and laws defined in Boolean algebra
Basics of digital electronics - I’m not talking going down to transistor level here, but you should be familiar with basic logical gates and how they are combined to form more complex circuits, like adders
Computer architecture - again, no need to go into details here. But, you should know and understand basic building blocks of CPU, how memory is organized and accessed, how data are represented inside computer (positive and negative number, floating point, character sets)
Basic blocks of programming languages - all programming languages have common structures. Learning them in language-independent way, and being able to recognize them, will make it easy for you to learn any programming language quickly. These building blocks include variables, operators, expression, statements, loops, conditionals and functions
2. Learn basic Linux usage
While you can program on any operating system (Windows, Mac OS X, Linux, BSD etc.), Linux has a special place in today’s software developmentt world.
With the advent of cloud computing, chances are you will deploy and run your applications in cloud server. And most of these servers are based on Linux, so being familiar with it will make your life much easier.
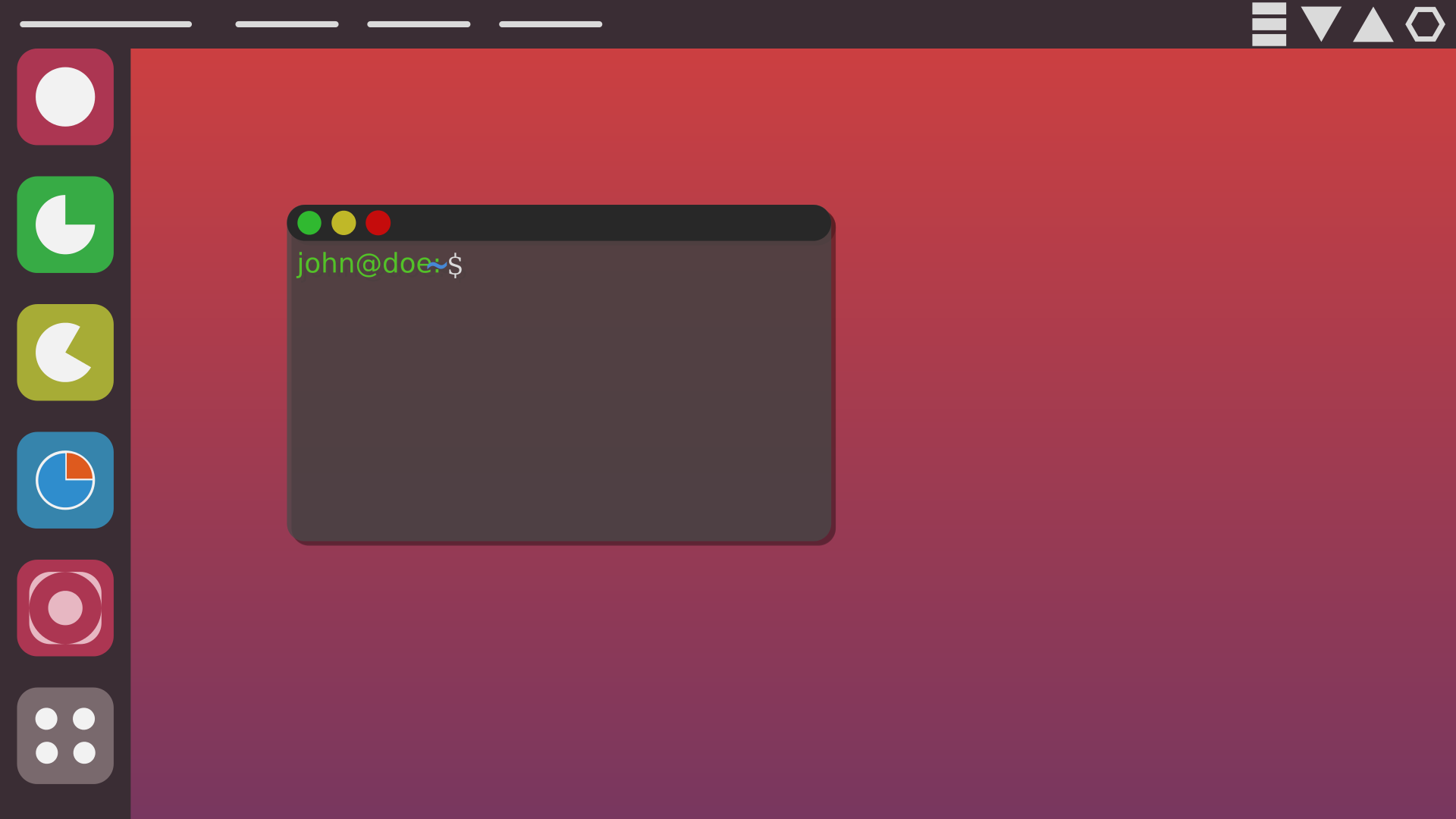
Learning Linux will give you exposure to working of servers and cloud
I’ve listed this step as the second in your path to learn programming, because it is important for the next step (as you will see).
While Linux is a full-blown operating system with a graphical user interface, you should focus on learning the shell and get comfortable working in it. As I said, you will most likely be deploying applications on Linux servers, and they always run without GUI.
You should learn the following:
Basic Linux commands for file manipulation - copy, move, read, edit files
Linux file system structure - get to know directory layout and which files go where. You will need this knowledge to know where to look for certain files
Working with archives and compression - learn about TAR, GZIP, BZ2 as most used archive formats. Get comfortable creating and extracting archives
Software management - learn to install, update and remove software packages. Get used to working with package managers, and also be comfortable installing software from source
Shell - get familiar with the concept of shell, environment variables, configuration files. Add new commands to PATH
Basic shell scripting - learn to write simple shell scripts to automate simple tasks. This would be your first taste of programming
3. C as your first programming language
Many people would be against recommending C as a first programming language, but in my opinion, it is THE perfect programming language for beginners.
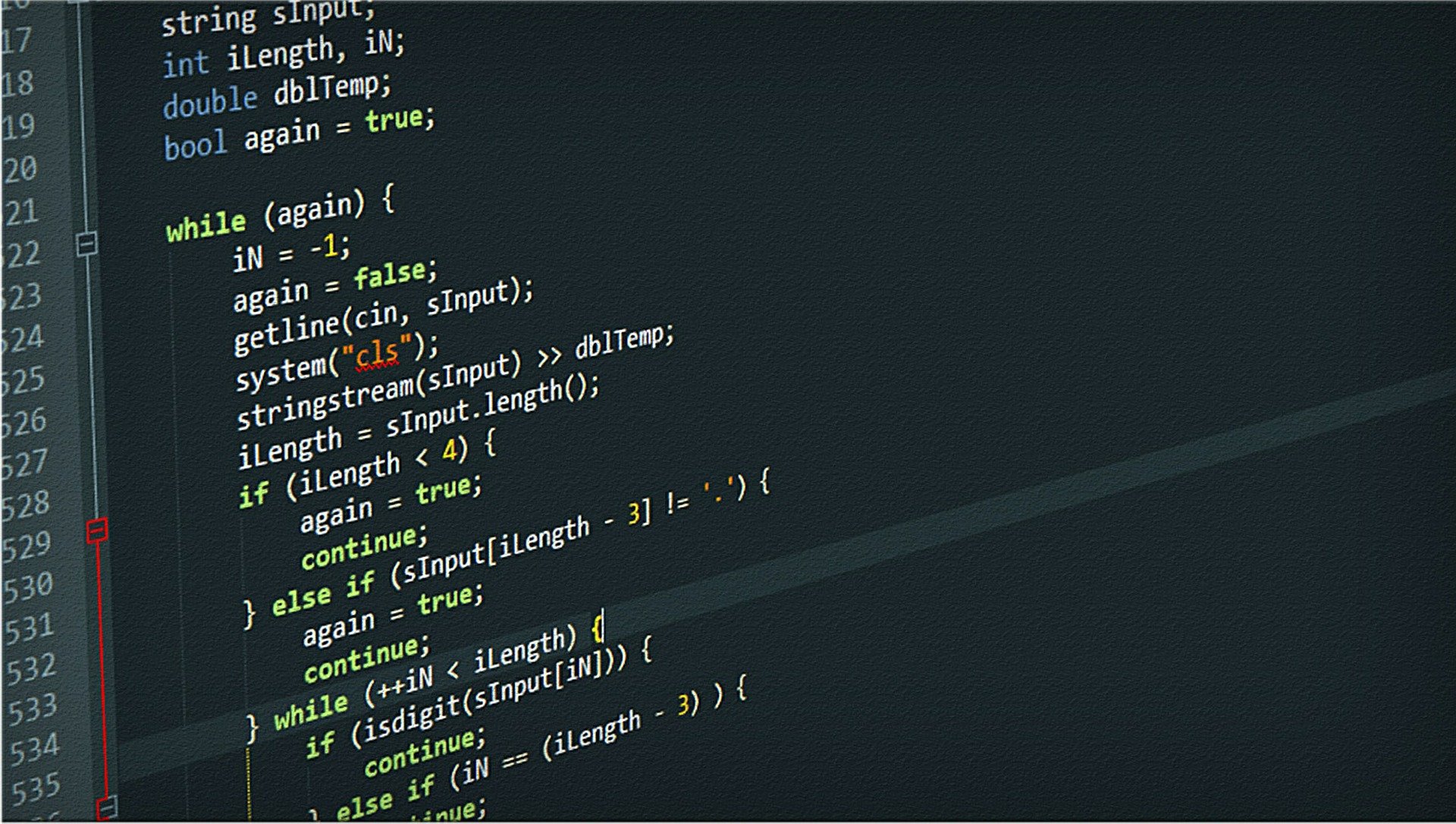
C is the best programming language for beginners
While languages like Python (which is mostly recommended as first language) are arguably easier, it is a double edged sword. They do make things easier, but at the same time, they hide some important concept which are really important for beginners to understand.
C, on the other hand, does not hide anything. You get exposure to the low levels details like memory management, raw strings, pointers… I believe it is really important for aspiring programmers to understand these things. In addition, after you learn C, you will be able to learn any language easily.
On top of that, C is the second most popular programming language, according to TIOBE. This means that there are a lot of job opportunities for C programmers.
You do not have to become a C guru, but you should be able to learn and understand the following:
basic data types and structures
working with pointers, arrays and strings
input/output, streams, reading and writing files, accepting user input
static and dynamic libraries, what they are and how to use them
be able to use compiler and linker, build software from source
4. Basic data structures and algorithms
Now that you have learned a programming language, it’s time to put it to good use. In this step, you should learn most common algorithms and data structure, and be able to implement them using C.
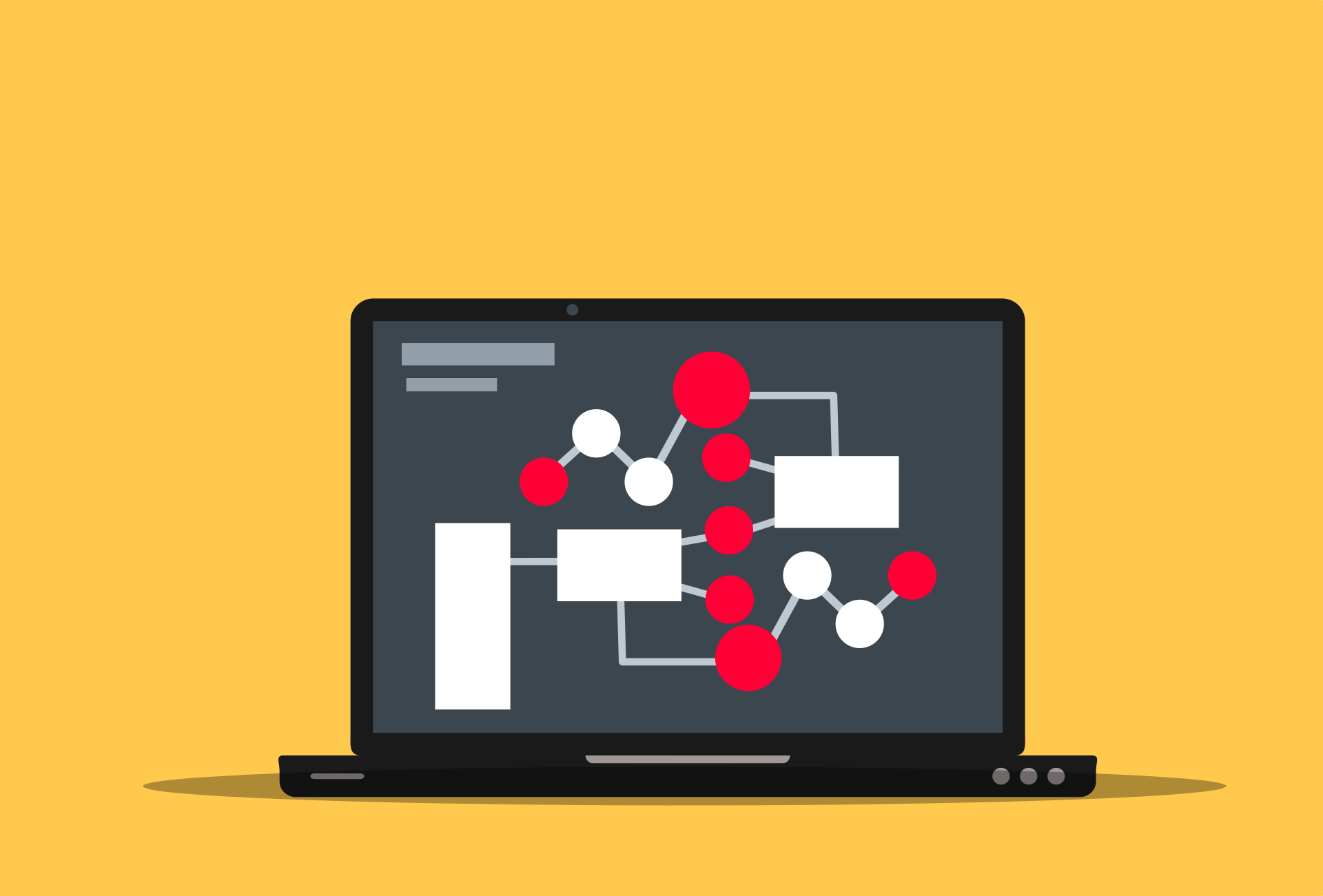
Basic data structures and algorithms
Here, the most important result is that you learn and understand runtime complexity of algorithms and data structures, and know when to use which. You’re not supposed to memorize properties of each one, but you should be able to tell which problems is best solved by using which algorithm.
In addition, you should be able to implement most commonly used on your own. This is great exercise, and something that is often asked at job interviews.
So, the first thing you should get familiar with is complexity analysis and Big O notation. Make sure you learn space and time complexity and how to evaluate complexity of your own implementations.
Next step is to learn the most commonly used data structures. I suggest you learn this before diving into algorithms, because knowing characteristics of data structures and when to apply them is critical. List of data structures to learn:
List - learn to implement a list backed by dynamic array and linked lists. Practice implementing singly and doubly linked lists.
Stack - implement your own stack with just basic operations, like
push
andpop
Queue - implement both single-ended and double-ended queue (where items can be picked up from both ends)
Hash table - when implementing this, you will also learn about hash functions
Heap - very useful structure for implementing sorting algorithms and priority queues
Tree - this is more general form of heap, and very good exercise to implement it
Now that you are familiar with data structures, you can get your feet wet with algorithms. Learn and implement the following algorithms:
Sorting algorithms - Bubble sort and insertion sort, as an introduction to sorting algorithms. After that, try some more advanced stuff like quicksort and mergesort. This will also give you exposure to recursion. Lastly, use the knowledge of heap to implement heapsort.
Binary search - after you know how to sort an array, you should be able to implement search function
Tree traversal - pre-order, post-order, in-order
5. Object Oriented programming with Java
Object oriented programming (OOP) is the most commonly used programming paradigm in today’s software development world. Despite the criticism and it’s shortcomings, it is by far the most important paradigm to learn.
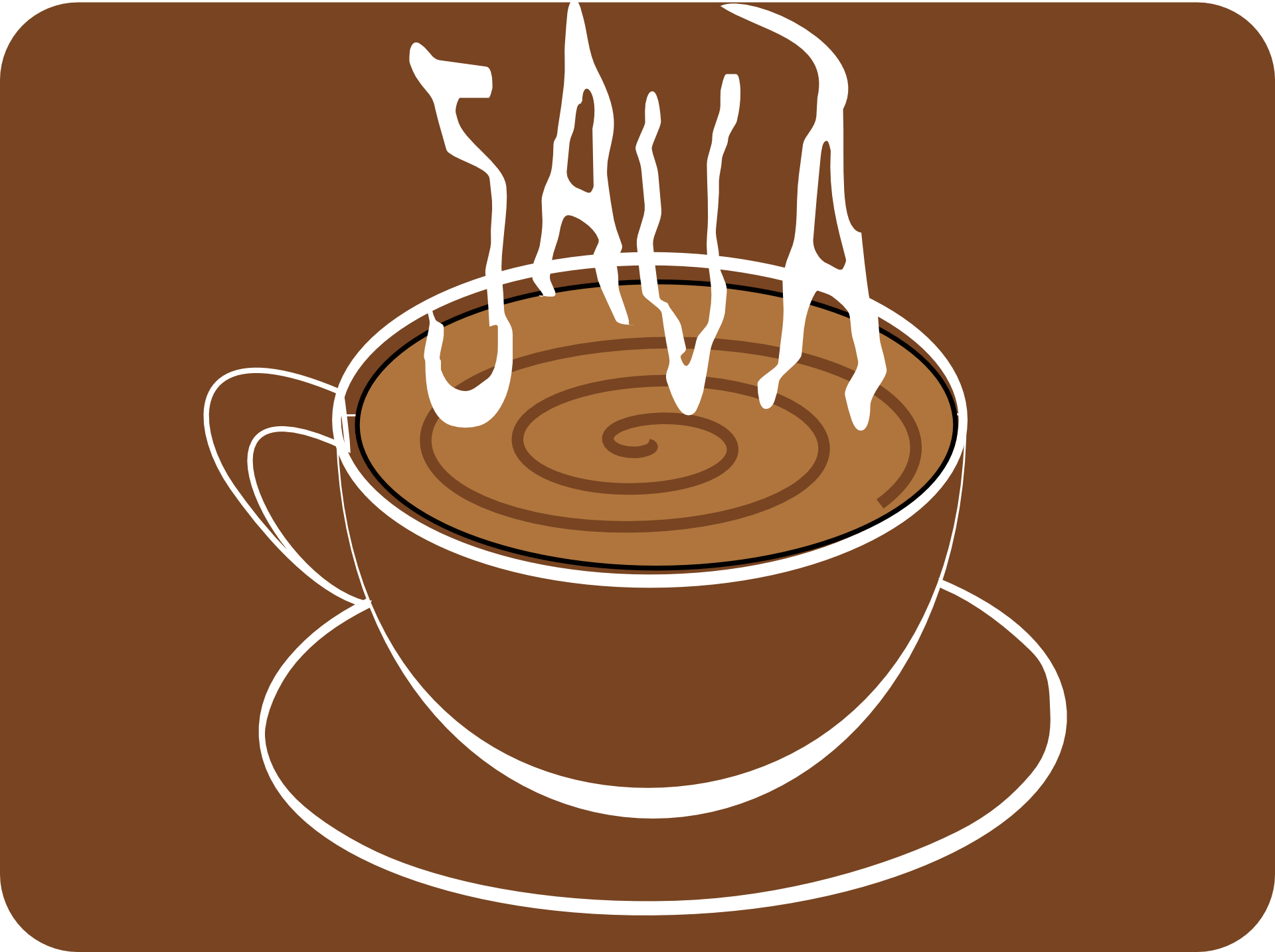
Object oriented programming with Java
As a companion to OOP, you should learn OOP-based language. Java is the best choice for this. It is fairly easy language to learn, it has huge ecosystem and community. In addition, it is one of the most popular languages in the world, with a wealth of jobs available. And, since Java is heavily based on C (which you learned in previous steps, right?), you shouldn’t have too hard time learning it.
As an added benefit, once you are comfortable with Java, you can easily pick up other JVM (Java Virtual Machine) based programming language. Some of the most popular are Kotlin, Scala and Clojure.
When learning object oriented programming, it is extremely important to understand 4 pillars of OOP:
Abstraction
Encapsulation
Inheritance
Polimorphism
In order to put this theory to good use, you will need to learn how these theoretical concepts are implemented in Java. You will need to learn and understand classes, interfaces, abstract classes, method overloading and overriding, type casting etc. This is in addition to basic programming concepts you learned in C (variables, loops, conditionals and the lot).
Finally, to get more proficient with Java, you should get to know it’s powerful standard library and use it for common programming problems. In addition, you should know common Java tools and how t use them:
Collections framework - common data structures for use with collections of data. This should also include Stream API
Multithreading API - learn to create and execute threads, synchronize them, share state and data between them
Input and output API - learn IO operations using both blocking and non-blocking APIs
Build tools - Maven is the de facto standard, so you should certainly learn it. If you feel like it, you should also get familiar with Gradle
at least one popular IDE (Integrated Development Environment) - IDE is practically a must for Java development and you should be proficient with it. Choose between InteliJ IDEA, Eclipse or NetBeans.
6. Networking fundamentals
In today’s interconnected world, it is hard to avoid writing software that doesn’t have ny network interaction. Most of applications today are either distributed, deployed to cloud or a number of servers, or talk to other network services.
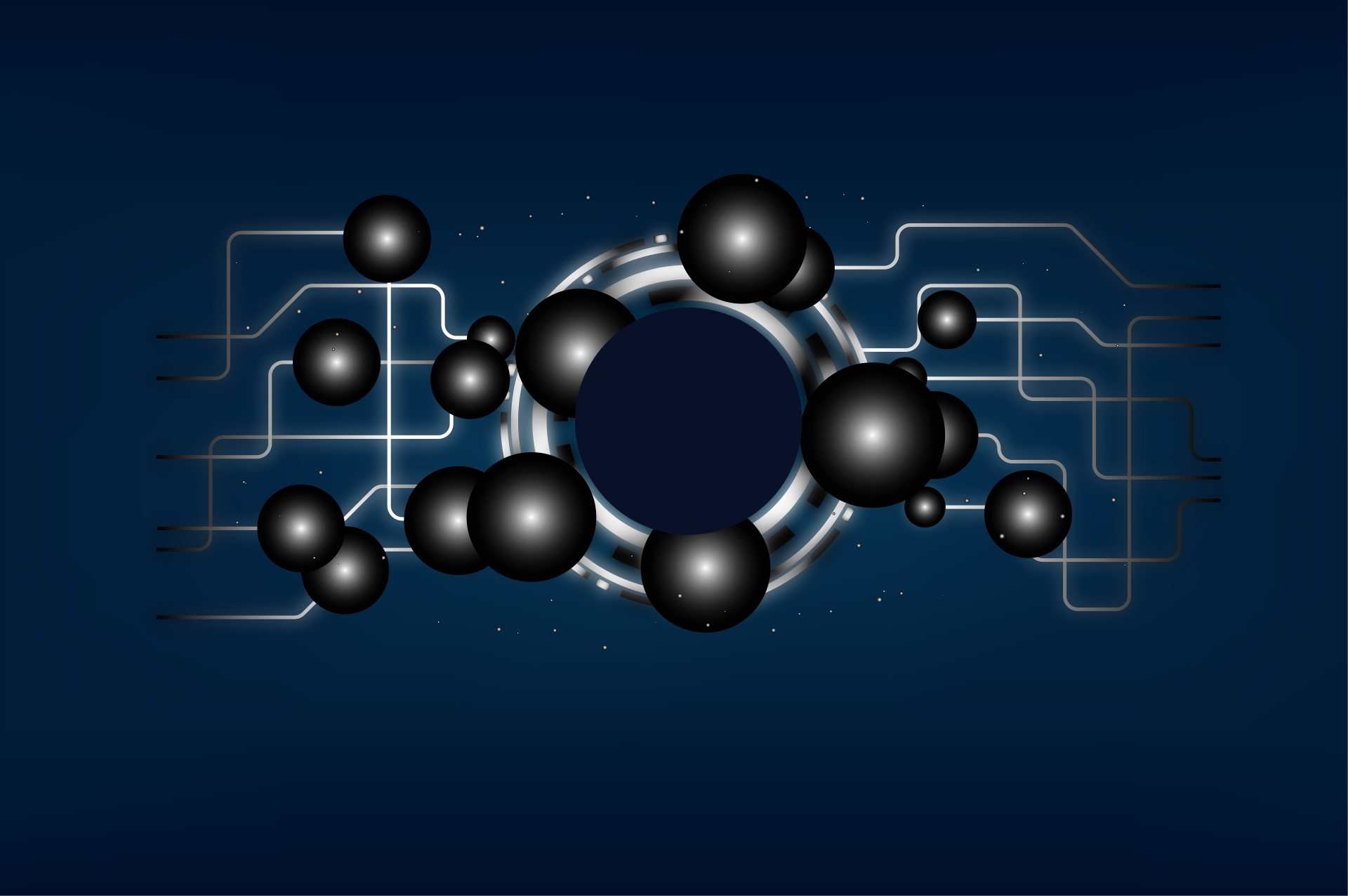
Get familiar with networking fundamentals
As a software developer, you don’t need to know the ins and outs of network protocols and low level details, but you should posses the basic understanding of network operations. You will need this knowledge to better understand why some things work the way they do, and it will tremendously help you with debugging network problems that your software might have.
For the beginner level, you should learn and understand the following:
OSI layers - be familiar with OSI networking stack, what is the responsibility of each layer and how they fit together
TCP/IP stack - this is by far the most widely used networking stack, and it is extremely important to know it well. You should be familiar with:
TCP vs UDP - kow the differences
IPv4 and IPv6 addresses, network mask, default gateway
subnetting networks, CIDR, network classes
what is port and socket
These two topics cover the basic network knowledge, but you should also be familiar with specific higher-level, widely used protocols. These include
DNS - the “phone book” of internet. You should be able to describe how DNS works and be able to create DNS records on your own
HTTP - this is by far the most widely used protocol in the internet. You don’t need to go into nitty-gritty details here, but you should know the request-response format, common headers, content negotiation, caching and security features
SSH - you should be able to connect to servers using SSH keys, copy files securely using SCP and configure client connections on your machine
This should be enough to get you ready for more complex development tasks using network resources.
7. Database basics
Almost every application needs a way to store data in some way. Most of the time, a database is used for that purpose. As a programmer, you need to be proficient with different kinds of databases.
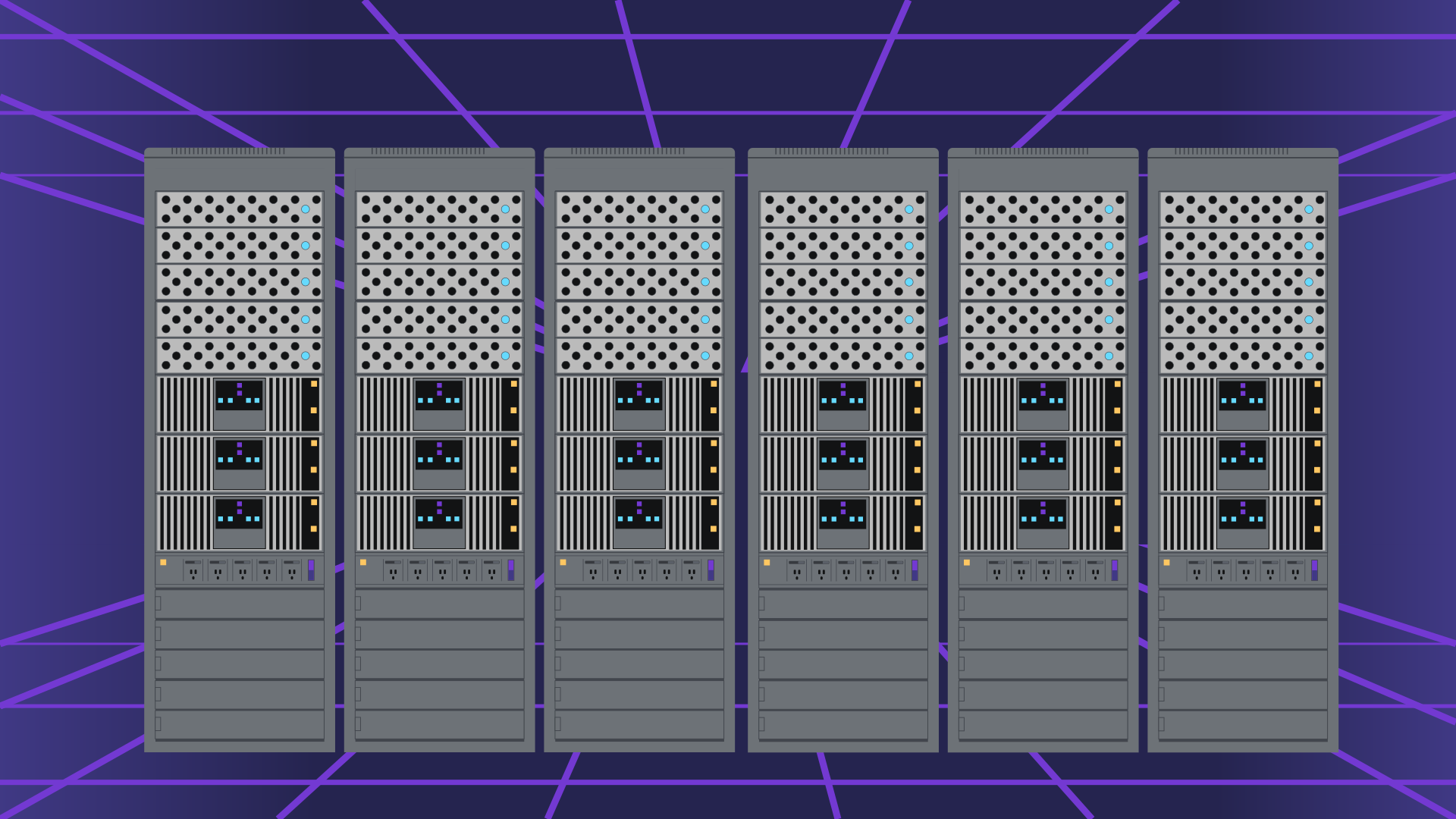
Learn the basics of database operation
Today, there are two flavors of databases, relational and non-relational (also known as NoSQL databases). With the advent of cloud computing and web applications, non-relational databases have become quite popular. But still, relational databases are the workhorse of software development.
In order to enhance your skills, you should be familiar with both kinds. Yet, my advice is that you focus on relational databases and SQL.
You should be familiar with the following topics regarding relational databases:
how data is organized in databases - tables, views, indexes
relations between data - primary key, foreign key, cardinality (one-to-one, one-to-many, many-to-many relations)
database design and normalization (first, second and third normal forms)
SQL basics - inserting, updating and deleting data, queries, joins, aggregation, grouping
get familiar with at least one database engine. A good choice is MySQL, which is one of the most popular databases in the world
Regarding non-relational databases, the situation is a bit different. There is no established standard, but rather NoSQL databases are suitable for specific use cases. There are document databases, key-valu databases, graph databases and so on. So, it is hard to pick one unless you know the exact use case you will be working on.
Maybe the best approach is to learn one NoSQL database to get familiar with the differences between relational and non-relational databases. For example, Mongo DB is good choice, since it is very popular and open source database.
8. Basic CI/CD concepts
Continuous Integration and Delivery (CI/CD for short) is a practice in software development where software is constantly built and deployed after every change. The main goal of CI/CD is to improve quality of code and speed of development by using automation. On every change to the code base, CI/CD pipelines build the code, run automated tests and deploy software.
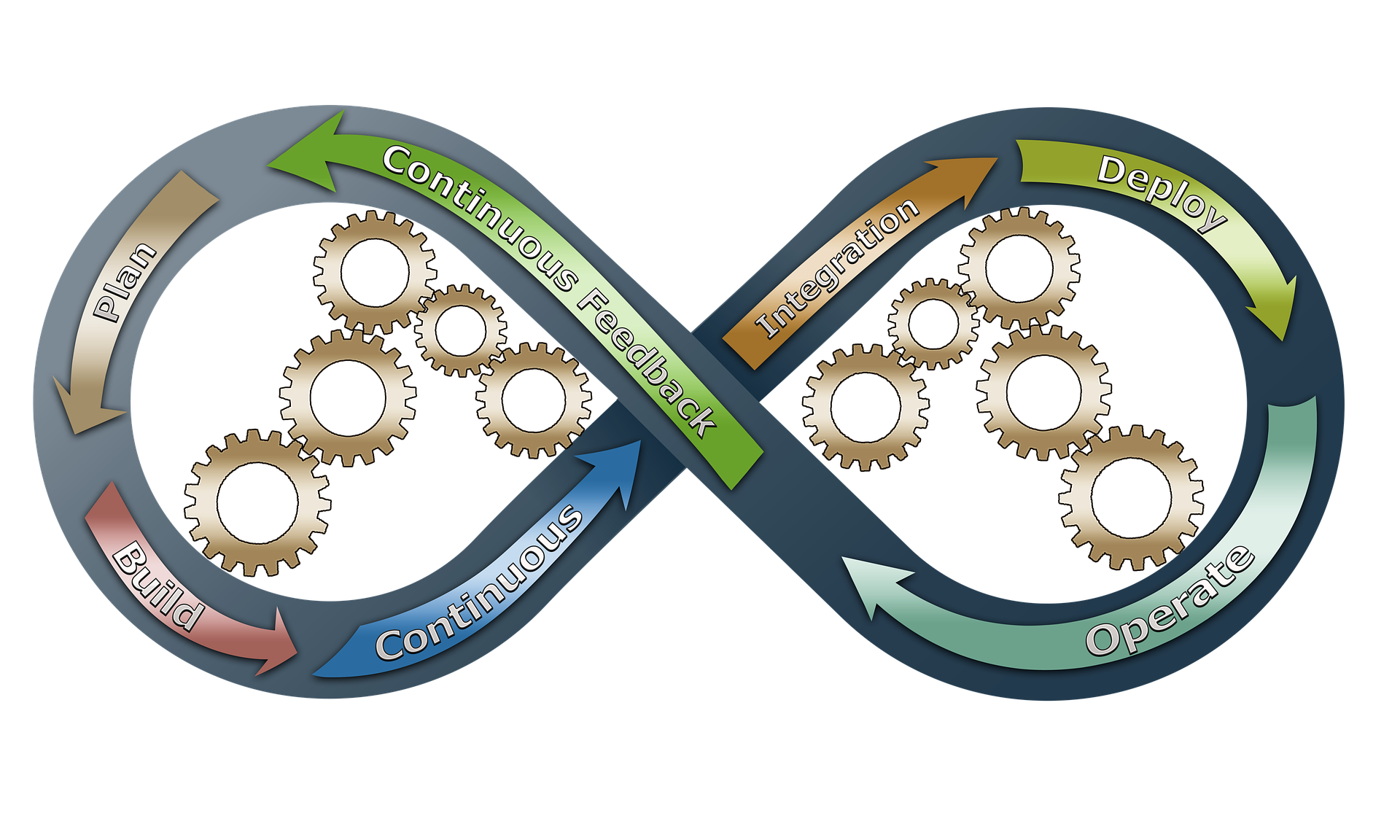
Although CI/CD is not exactly part of software development, good programmer should be familiar with the basic concepts and approaches to this practice. You should be able to setup simple CI/CD pipelines to build and deploy your code.
As part of this step, you should learn about the following:
working with git - learn to setup a repository, configure hooks to trigger builds, creating and merging pull requests
learn at least one CI tool. Good starting point is Jenkins, a popular open source CI tool. It has great support for many languages and build tools, and you can use it with almost any language
working with artifact repositories - artifact repositories are where you store the output of the build. Final step of the build is to deploy build artifact to repository. Depending on which programming language you use, you will be working with various types of repositories. Learn the one that is mostly used with your programming language.
9. Security best practices
As the software become more and more prevalent, it increasingly becomes the target of hackers cyber criminals. It is estimated that damages from cyber crime will [reach $$10.5 trillion](Cybercrime To Cost The World $10.5 Trillion Annually By 2025) by 2025.
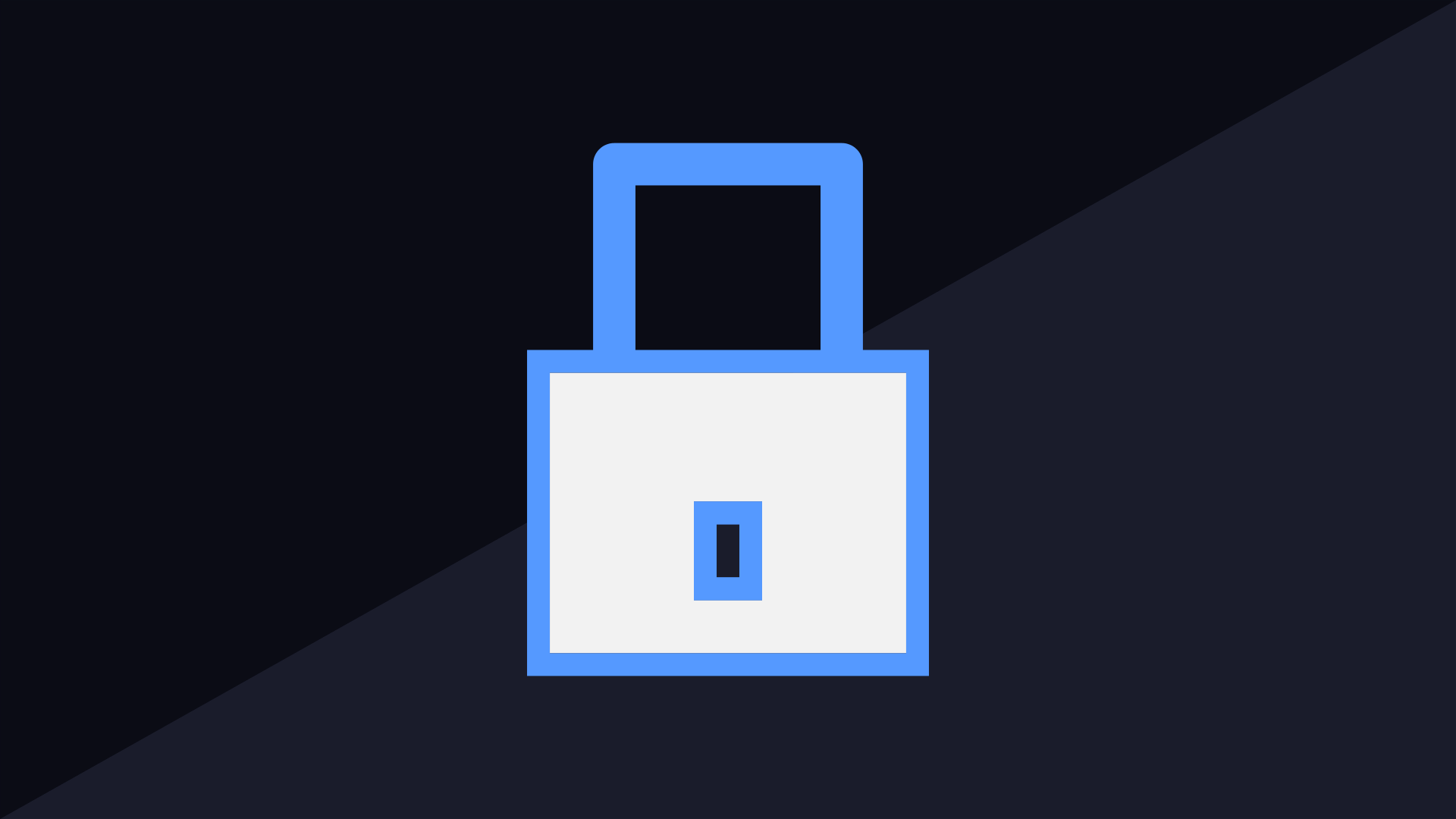
With this in mind, it is imperative that software engineers take great care to mitigate well know threats and vulnerabilities. While it is impossible to make software bullet-proof, following the recommended best practices makes for a smaller attack surface, and less possibility for error.
A beginner developer should be familiar with the following common security threats and how to avoid them:
Cross site scripting (XSS)
SQL injection
Clickjacking
Buffer overflow
Good source for information about security threats and how to avoid them is OWASP foundation. You can find here the list of security best practices at the different parts of software.
One more area that is very important for developers is working with digital certificates, SSL/TLS and public key infrastructure. As a developer, you will be using certificates and key all the time, and you should have solid understanding of how they work.
Getting familiar with security best practices will make you a much better programmer.
10. Virtualization and cloud
Virtualization has become important part of software development and deployment. The best example is cloud, which is essentially large-scale virtualization platform for running virtual machines and containers.
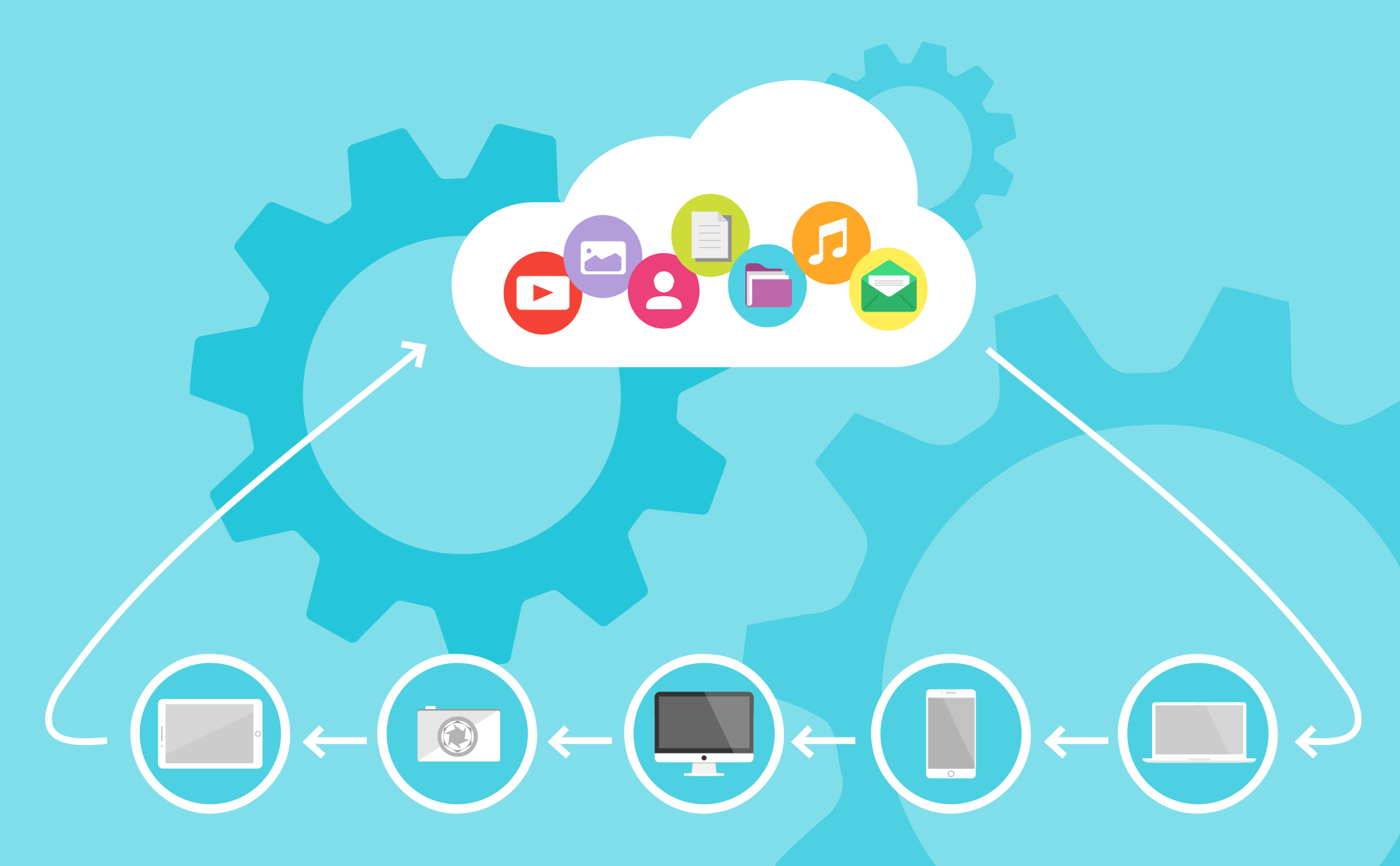
There are many virtualization platforms out there, and knowing details of any of them is not really a requirement for most software developers. But, in the process of learning programming, you should get familiar to using at least widely popular platforms.
In a nutshell, you should learn the following:
a hypervisor for running virtual machines. For this case, Virtualbox is good starting point. It is free, open source, multi platform software package. You can install it on your development machine and launch virtual machines with different operating system
container platform - there are many platforms for running containers, but Docker is the de-facto standard. You should learn how to create Docker container images, launch them, expose ports, creating networks. In addition, as a tool to orchestrate containers, you should be familiar with [Docker Compose](Overview of Docker Compose | Docker Documentation).
at least one public cloud provider - the big three are AWS, Azure and Google Cloud Platform. My recommendation here is AWS, since it is the most popular of the three. AWS has a free tier, where you can test most of the services free of charge for 12 months.
If you are new to cloud, take a look at this introduction to cloud computing post. It is geared toward beginners who want to understand basics of cloud computing.
Final thoughts
There you have it, entire curriculum to learn programming laid out for you! At the first glance, it might seem intimidating, but as you follow through, you will notice that each step builds upon the previous. It makes for the logical progression which should take you to the final goal: getting employment as software developer.
Now, notice that I did not speak of any specific area of software development, such as frontend, backend, mobile and so on. This is because this approach is intended to get you up to speed with basics. Once you learn the concepts outlined in this post, you should have no problems getting into any area of programming you want to.
I would love to hear your thoughts on this. If you have any questions, comments, opinions, just send a comment using a form bellow. I would be glad to answer to any comment.