Java Static Code Analysis Tools
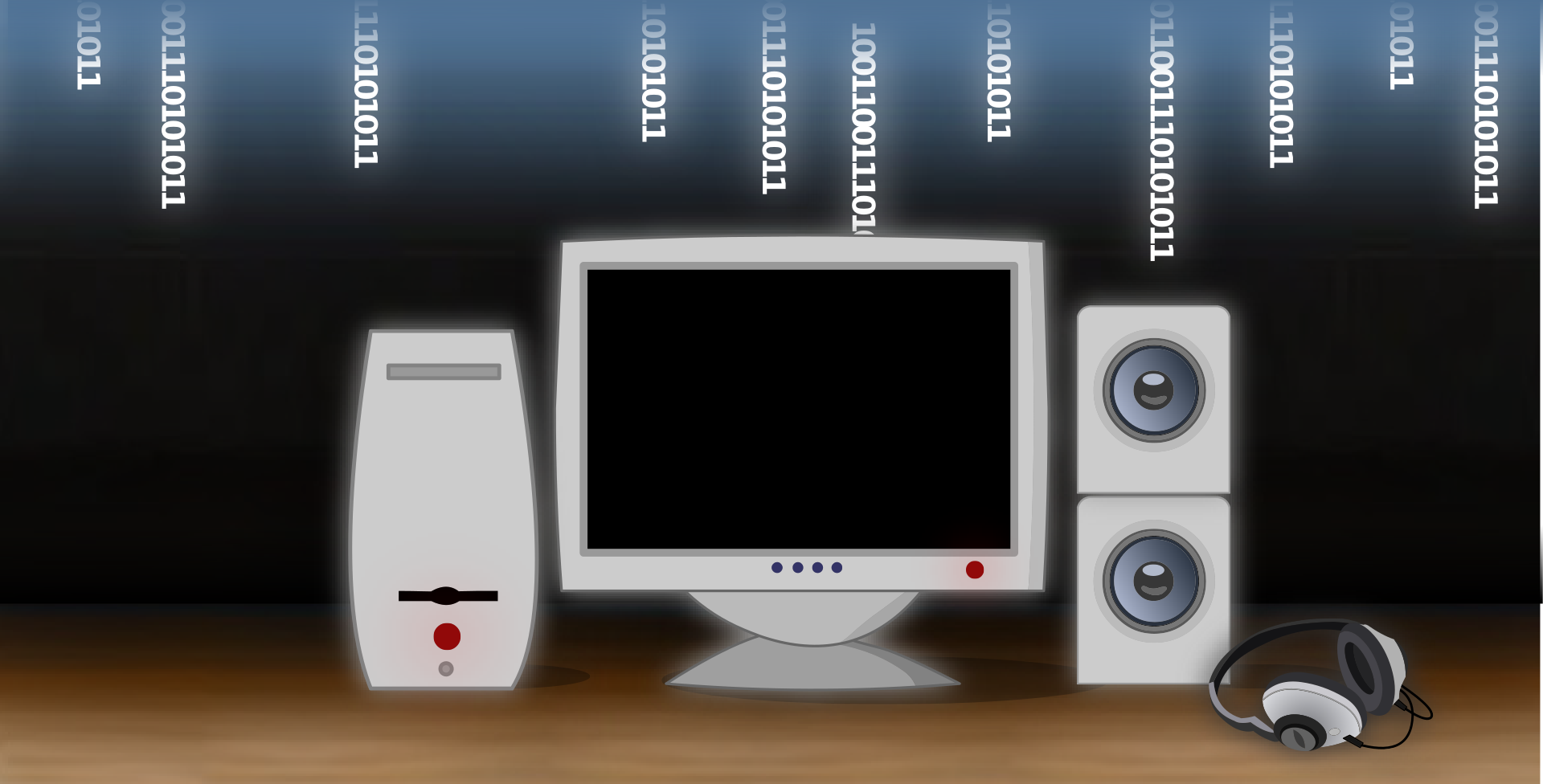
Static Code Analysis is a part of software development process intended to improve overall quality of source code. It scans source code for any known pattern that can lead to potential bugs, security vulnerabilities and bad practices. It is a part of overall code quality improvement process.
In this post, I will present some of commonly used tools for static code analysis, specifically targeted to java projects. You can find complete code for sample in github repo.
Table Of Contents
Checkstyle
Checkstyle is a tool that enforces predefined code formatting rules for Java source code. Code formatting is important for large projects with many developers to make sure that source code is consistent and compliant with standards.
Checkstyle provides a Maven plugin, so it can be easily integrated with any Maven project. It can be configured to enforce custom rules, but comes by default with Sun Code Conventions rules, which are widely used in java projects. It is easy to customize and add your own rules.
Adding Checkstyle to your Maven project is as easy as configuring a plugin in your POM’s pluginManagement
section:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.1.2</version>
</plugin>
Sample project contains couple of classes with intentional violations to showcase how Checkstyle works.
Generating analysis report
You can generate a report for project by running a simple command in project root directory:
mvn checkstyle:checkstyle
This will generate report XML file in target/checkstyle-report.xml
. Additionally, it will generate more human readable HTML report in target/site/checkstyle.htm
.
Sample HTML report is shown in the following image:
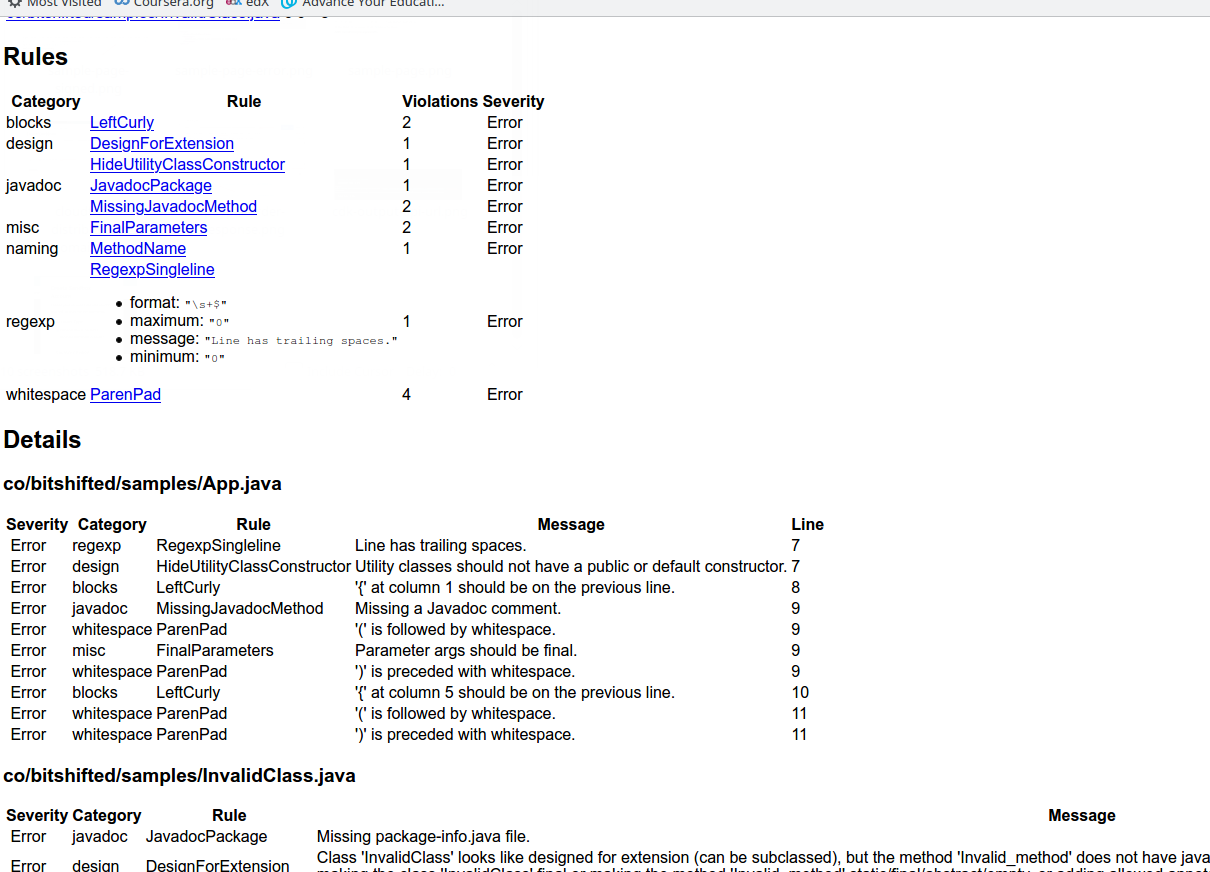
Checkstyle HTML report
Linking to source code
As you can see, generated HTML report is pretty bare-bones. For every reported violation, you need to find a file and line where it is in order to fix it. This ca get tedious real fast.
Fortunately, there is a way to make the report more user friendly. We can add links to source code, so we can get a better overview of reported violations.
In order to do this, we need to configure maven-jxr-plugin
. This plugin will create HTML version of source code to which violation reports can link. We need to add the following in POM file:
<pluginManagement>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jxr-plugin</artifactId>
<version>3.2.0</version>
</plugin>
</pluginManagement>
.........
<reporting>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jxr-plugin</artifactId>
</plugin>
</plugins>
</reporting>
When you now run the command above, you will notice that violations have links next to them which lead to source code:
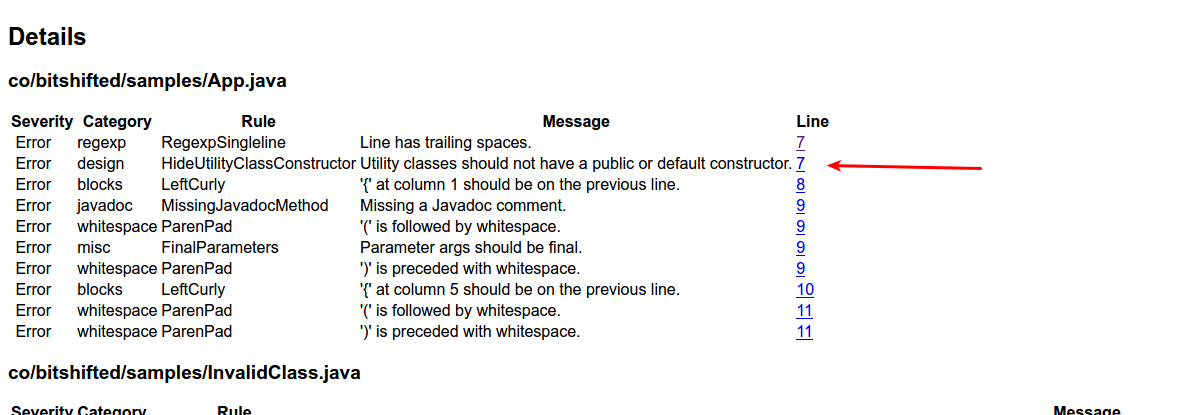
Checkstyle HTML report with links to source code
Enforcing the code style rules
Best approach to code analysis is to run it automatically as part of automated build pipeline. In this case, you probably want to fail the build if there are violations. In order to enforce this, you can configure Checkstyle plugin to be run as a phase of the build:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<configuration>
<consoleOutput>true</consoleOutput>
<failOnError>true</failOnError>
</configuration>
<executions>
<execution>
<id>validate-code</id>
<phase>validate</phase>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
This configuration will run Checkstyle during build validation phase. If any errors are found, the build will fail.
Spotless
Spotless Maven plugin is in many ways similar to Checkstyle. Main difference is that it’s more generic, has more configuration options and supports more languages.
One big advantage that Spotless has over Checkstyle is that it has an apply
command which allows you to fix all violations with one stroke. Compared to Checkstyle, in which you need to fix them all manually, this is a great improvement.
If you have a large project where you want to introduce formatting rules, Spotless can be big help. Using it, you can make whole project rules-compliant in one swoop.
PMD
PMD is another static code analysis tool. While Checkstyle and Spotless focus on formatting, PMD is more concerned with enforcing programming best practices and finding common programming flaws.
It also has a Maven plugin which allows you to easily incorporate it in your Maven projects:
<reporting>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-pmd-plugin</artifactId>
<version>3.16.0</version>
<configuration>
<rulesets>
<ruleset>/rulesets/java/braces.xml</ruleset>
<ruleset>/rulesets/java/naming.xml</ruleset>
</rulesets>
</configuration>
</plugin>
</plugins>
</reporting>
Note that we have specified some rules that we want PD to check. You can learn more about PMD rule sets .
You can generate the report by running the following command:
mvn pmd:pmd
This will generate the report in target/site/pmd.html
file. Report looks like the following screenshot:
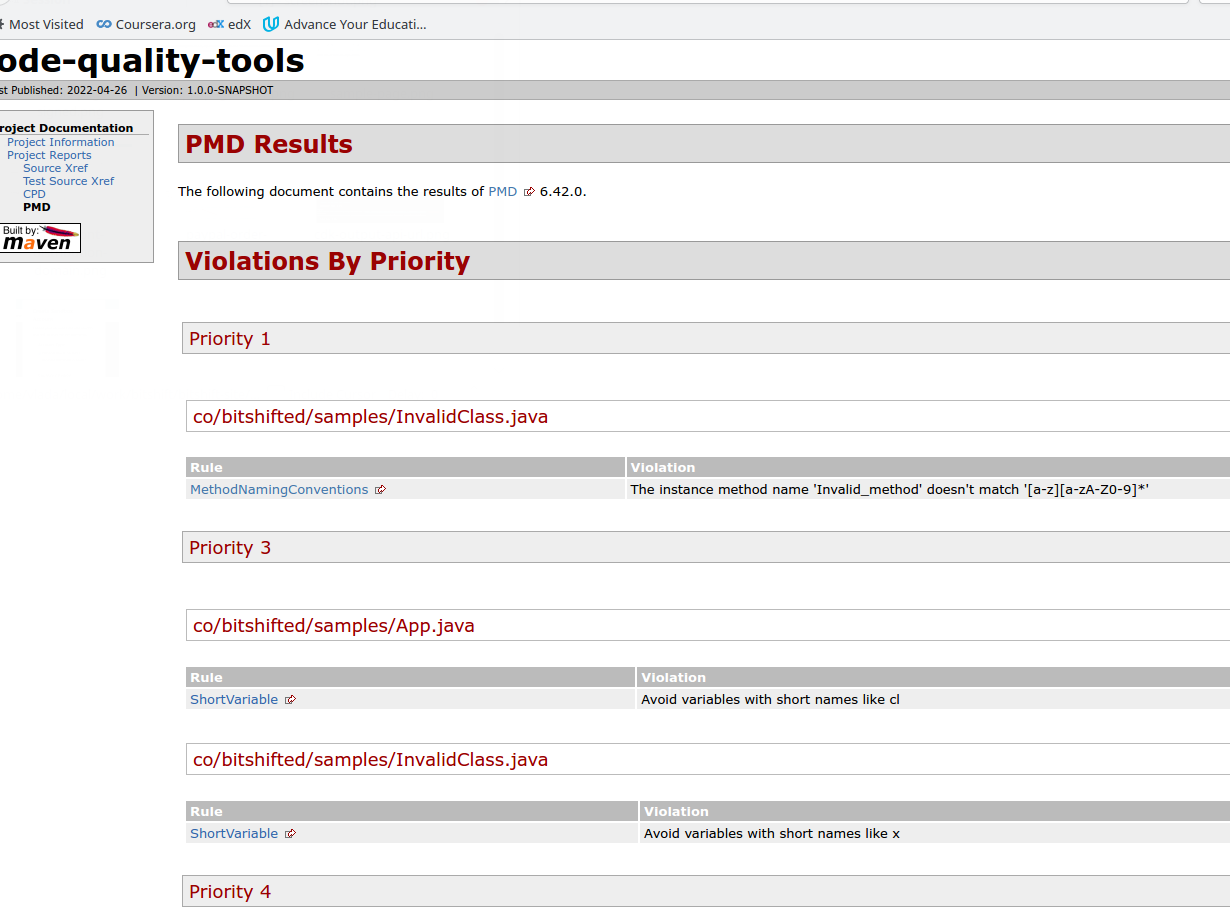
PMD generate HTML report
SpotBugs
SpotBugs is a static analysis tool that helps find potential bugs in Java code. It is a fork of FindBugs project, which has been discontinued.
SpotBugs looks for more than 400 known bug patterns in your source code. You can find a list of supported bug patterns here.
To configure SpotBugs, add it as a build plugin to your POM file, like so:
<build>
<plugins>
<plugin>
<groupId>com.github.spotbugs</groupId>
<artifactId>spotbugs-maven-plugin</artifactId>
<version>4.5.3.0</version>
</plugin>
</plugins>
</build>
To generate a report, run the following command:
mvn spotbugs:spotbugs
SpotBugs generates report in XML format. This can be difficult to read, so SpotBugs provides a GUI tool to view the result. You can launch the tool by running command mvn spotbugs:gui
.
The following screenshot shows running GUI with generated report:
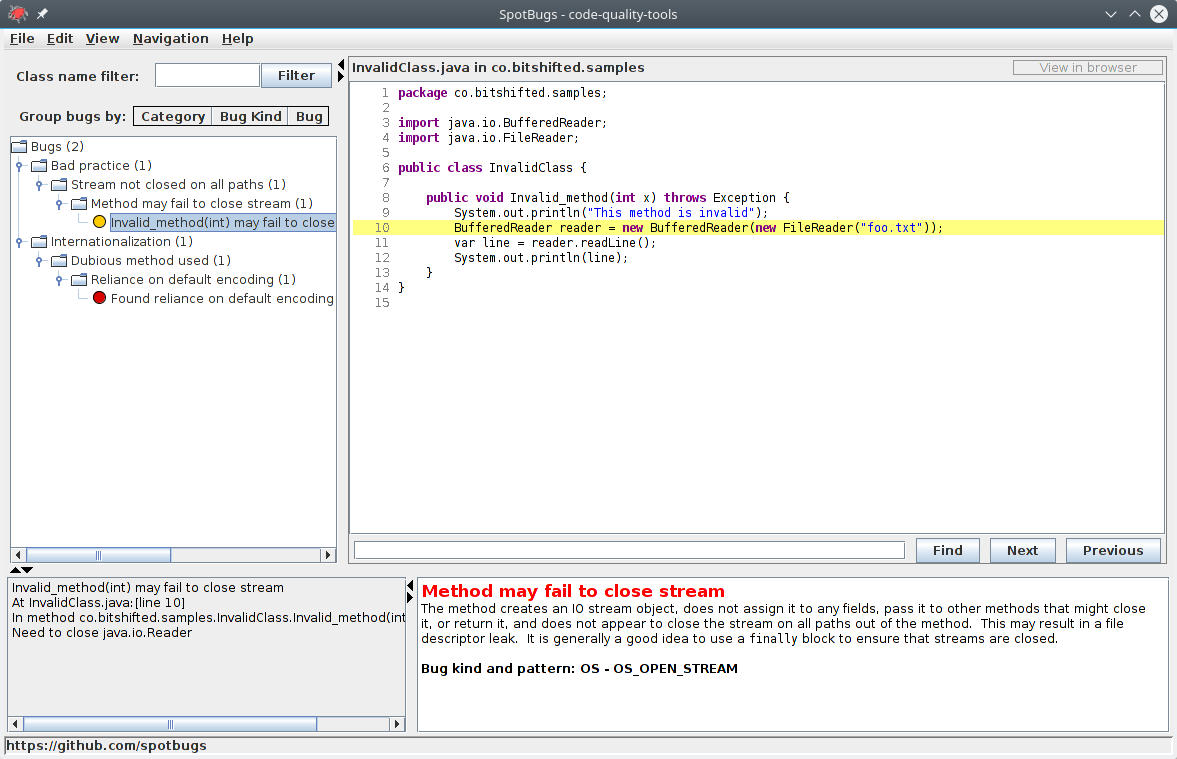
SpotBugs GUI showing generated report
Just like other plugins we’ve seen, this one can be configured to fail the build if any errors are found.
Final thoughts
In this port, I’ve listed most commonly used tools for static analysis of Java code. Using these can greatly improve quality of your code.
If you are using automated CI/CD pipelines (and you are using it, right? ;-) ), it might be a good idea to use a tool like SonarQube. It integrates all the tools we mentioned, but provides additional capabilities, such as trends, issues tracking, user friendly UI and much more.
If you have any questions and comments about this, feel free to post a comment using a form bellow.